Programming Language Syntax Highlighting
You can use indented and fenced code blocks for programming language syntax highlighting on your canvas. Create snippets and utilize copy to clipboard functionality to easily utilize your repeating actions. Copy to clipboard button is available on the right hand side of the code block.
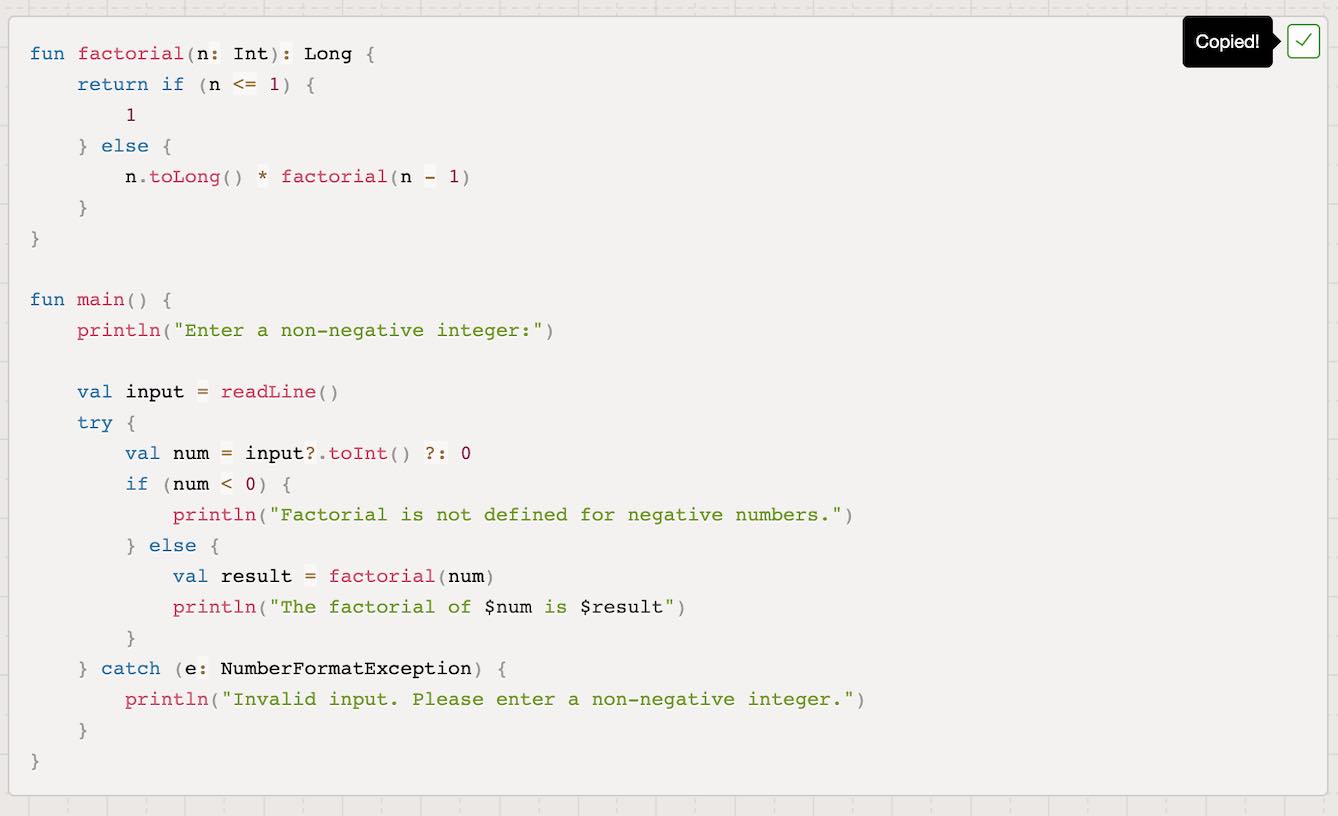
Sketchboard supports several programming languages for syntax highlighting:
- JavaScript - (js)
- TypeScript - (ts)
- Go programming language - (go)
- Scala - (scala)
- Python - (python)
- Java - (java)
- Kotlin - (kotlin)
- Clojure - (clojure/clj)
- Rust - (rust)
- Swift - (swift)
- CSharp - (csharp)
- C++ - (cpp)
- Haskell - (haskell)
Other file content highlighting
- SQL - (sql)
- JSON - (json)
- XML - (xml)
- HTML - (html)
- Shell - (bash/shell)
- Makefile - (makefile)
- YAML - (yaml)
Use a sequence of at least three consecutive backtick characters (`) or tildes (~). (Tildes and backticks cannot be mixed.) Check out some of the examples of using syntax highlighting with the notes shape below.
Example shell script syntax highlighting.
~~~shell
mkdir -p /tmp/foo
~~~
Which renders on Sketchboard:
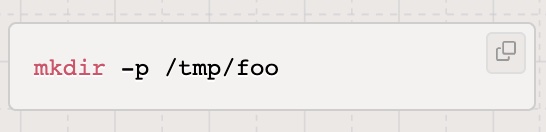
Let us know if your favorite programming language is missing!
JavaScript
Example code snippet:
~~~java
class Foo {
public static void main(String[] args) {
}
}
~~~
Unrendered and rendered look on Sketchboard
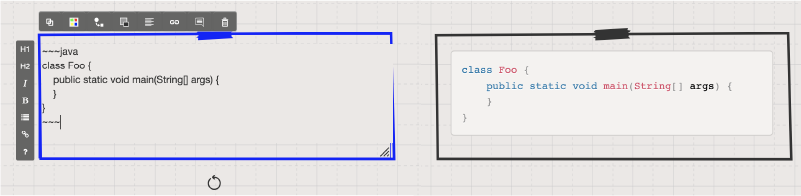
Go Programming Language
Example code snippet:
~~~go
type Abs struct {
Field string `json:"field"`
}
func main() {
}
~~~
Unrendered and rendered look on Sketchboard
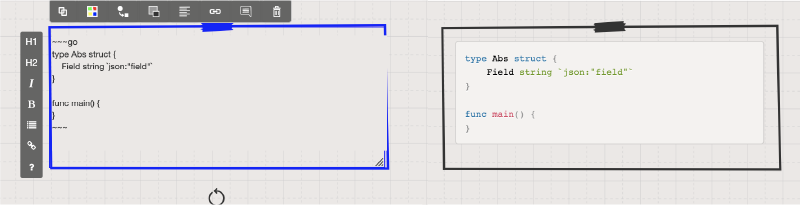
Scala
Example code snippet:
~~~scala
object BoardSnippet extends BoardUtils with PreviewFileHelpers with LastAccess with SLoggable {
private def parseAndCheck(
item: JValue,
secCheck: (Diagram) => Boolean
): Box[Diagram] = {
for {
board <- JsonBoardInfo(item)
diagram <- Diagram.findWithAccountByName(board.boardId)
if secCheck(diagram)
} yield {
diagram
}
}
}
~~~
Unrendered and rendered look on Sketchboard
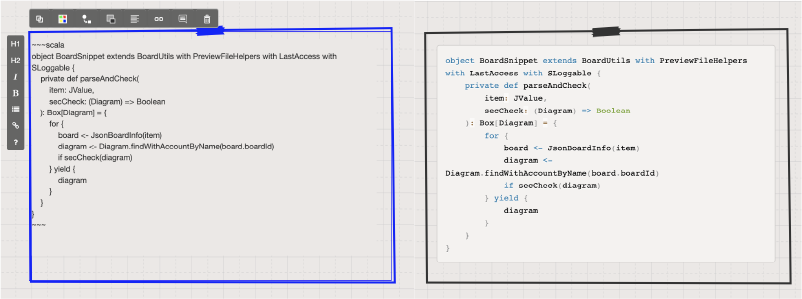
SQL
Example code snippet:
~~~sql
SELECT * FROM accountuser au JOIN account a ON a.id = au.account;
~~~
Unrendered and rendered look on Sketchboard

HTML
Example code snippet:
~~~java
class Foo {
public static void main(String[] args) {
}
}
~~~
Unrendered and rendered look on Sketchboard
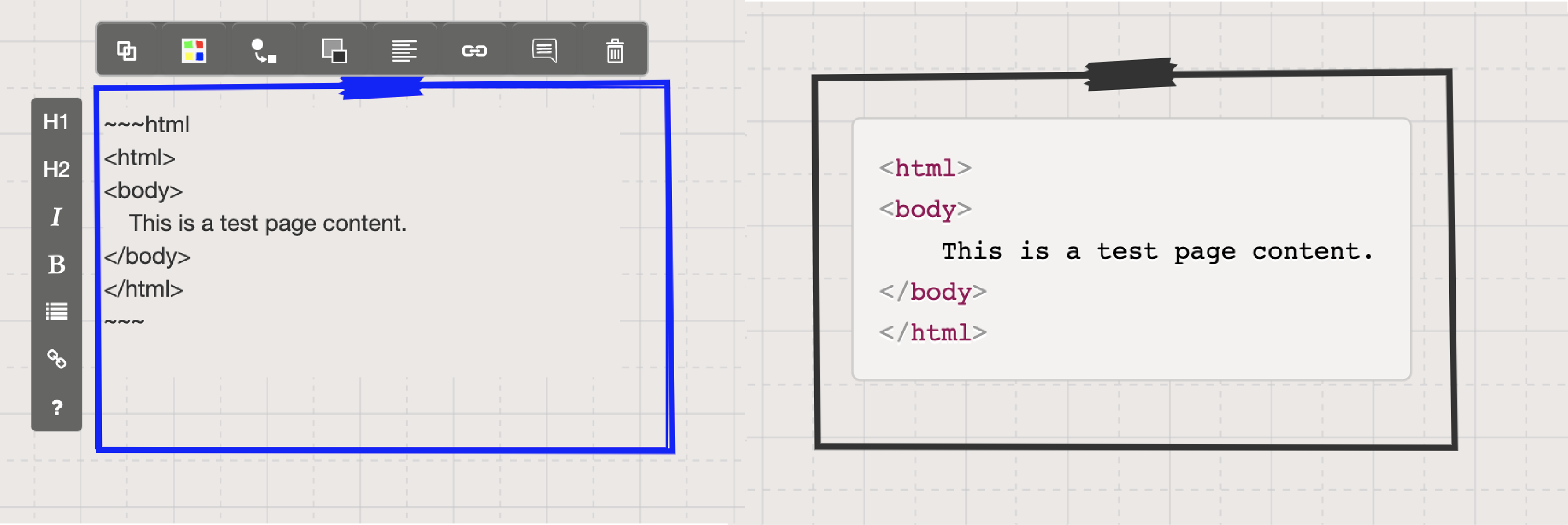
JSON
Unrendered on Sketchboard
Rendered
Kotlin
~~~kotlin
fun factorial(n: Int): Long {
return if (n <= 1) {
1
} else {
n.toLong() * factorial(n - 1)
}
}
~~~~
Rendered:
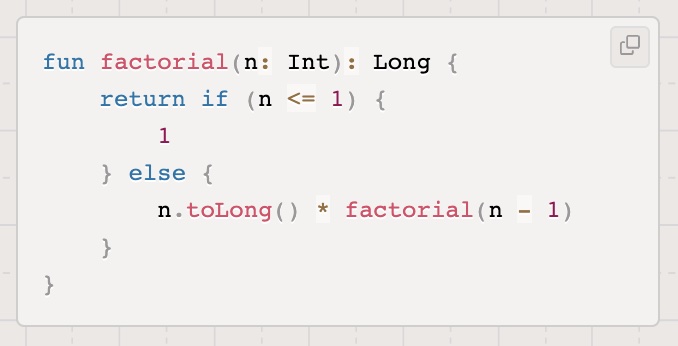
Clojure
~~~clj
(ns command-line-demo.core
(:gen-class))
(defn -main [& args]
(if (empty? args)
(println "Usage: clj -m command-line-demo.core [arg1 arg2 ...]")
(println (str "Hello, " (apply str args) "!"))))
~~~
Rendered:
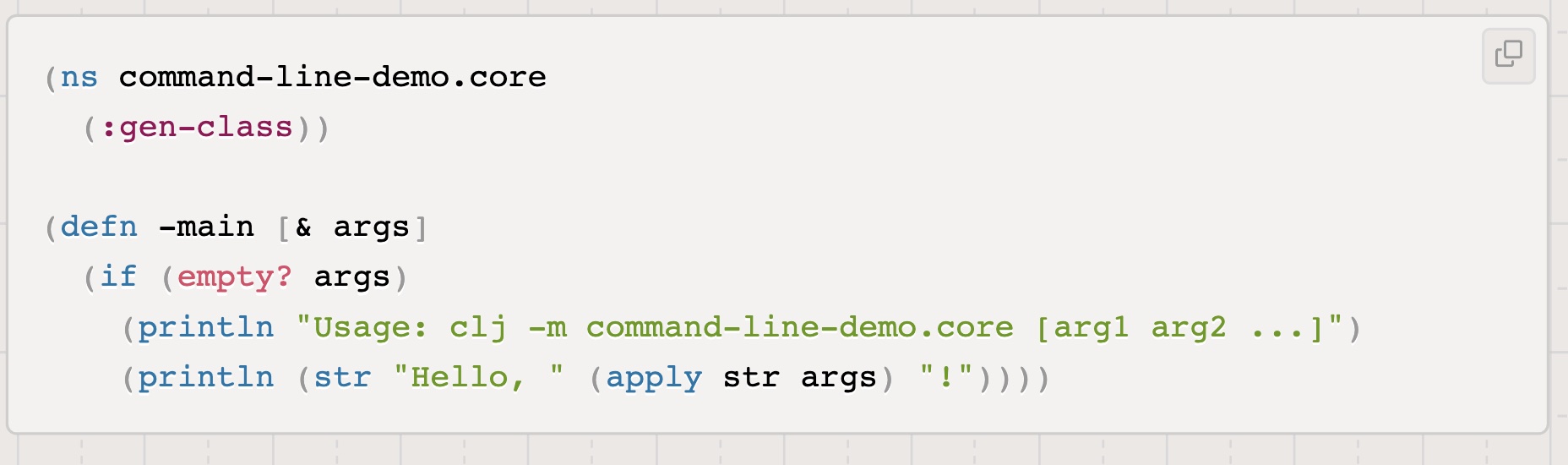
Rust
~~~rust
use std::io;
fn is_palindrome(input: &str) -> bool {
let reversed: String = input.chars().rev().collect();
input == reversed
}
fn main() {
println!("Enter a word or phrase:");
let mut input = String::new();
io::stdin()
.read_line(&mut input)
.expect("Failed to read line");
let cleaned_input = input.trim().to_lowercase();
if is_palindrome(&cleaned_input) {
println!("It's a palindrome!");
} else {
println!("It's not a palindrome.");
}
}
~~~
Rendered:
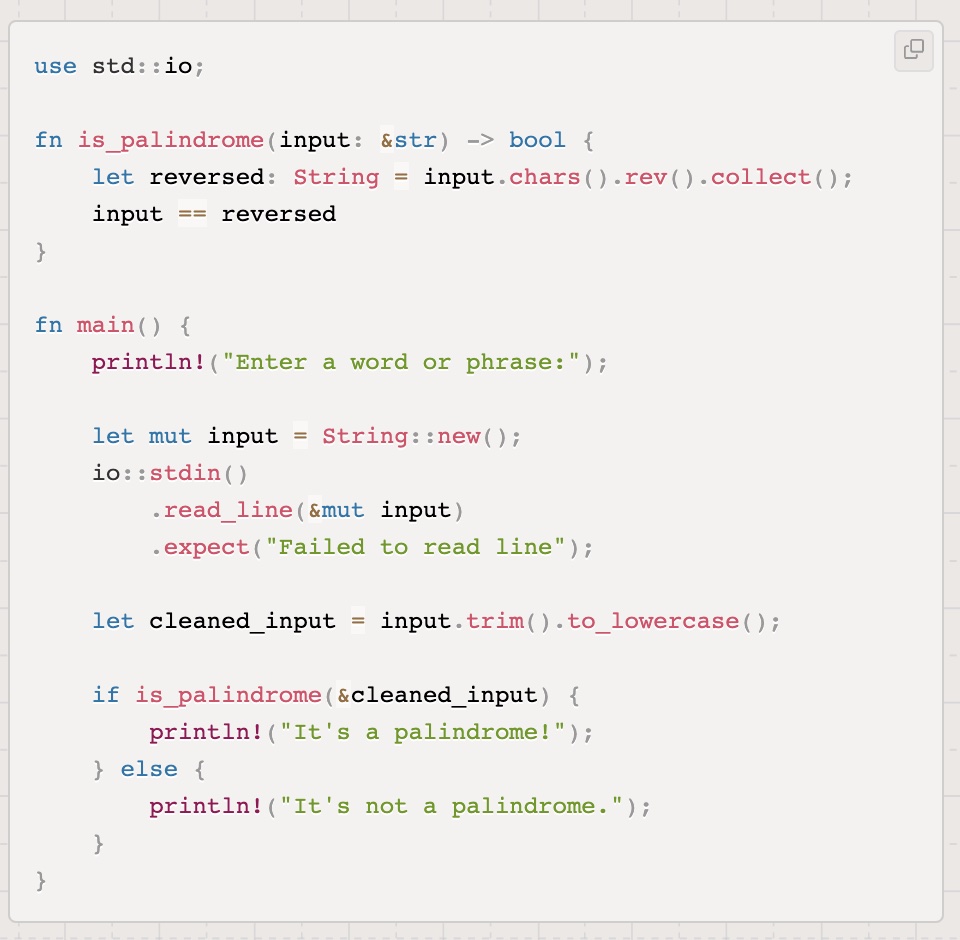
Swift
~~~swift
func sumOfEvenNumbers(upTo n: Int) -> Int {
var sum = 0
for number in 1...n {
if number % 2 == 0 {
sum += number
}
}
return sum
}
let n = 10 // Change this to the desired positive integer
let result = sumOfEvenNumbers(upTo: n)
print("The sum of even numbers from 1 to \(n) is \(result)")
~~~
Rendered:
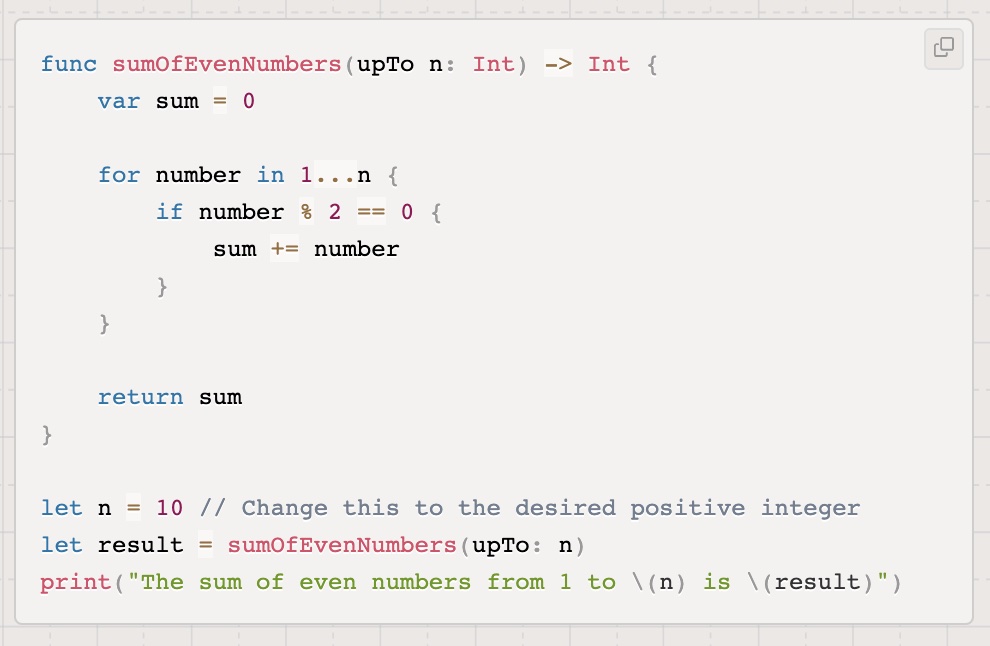
Once you have the syntax highlighted shapes on your Sketchboard canvas, you can also create additional shapes to initiate conversation about the code. Check out our blog post about how to use syntax highlighting here.
Escaping
When you want to show characters used in formatting, those needs to be
escaped with \.